The Broad Life - Your Ultimate Guide to Finding Duplicate Characters in a String in C Programming Language
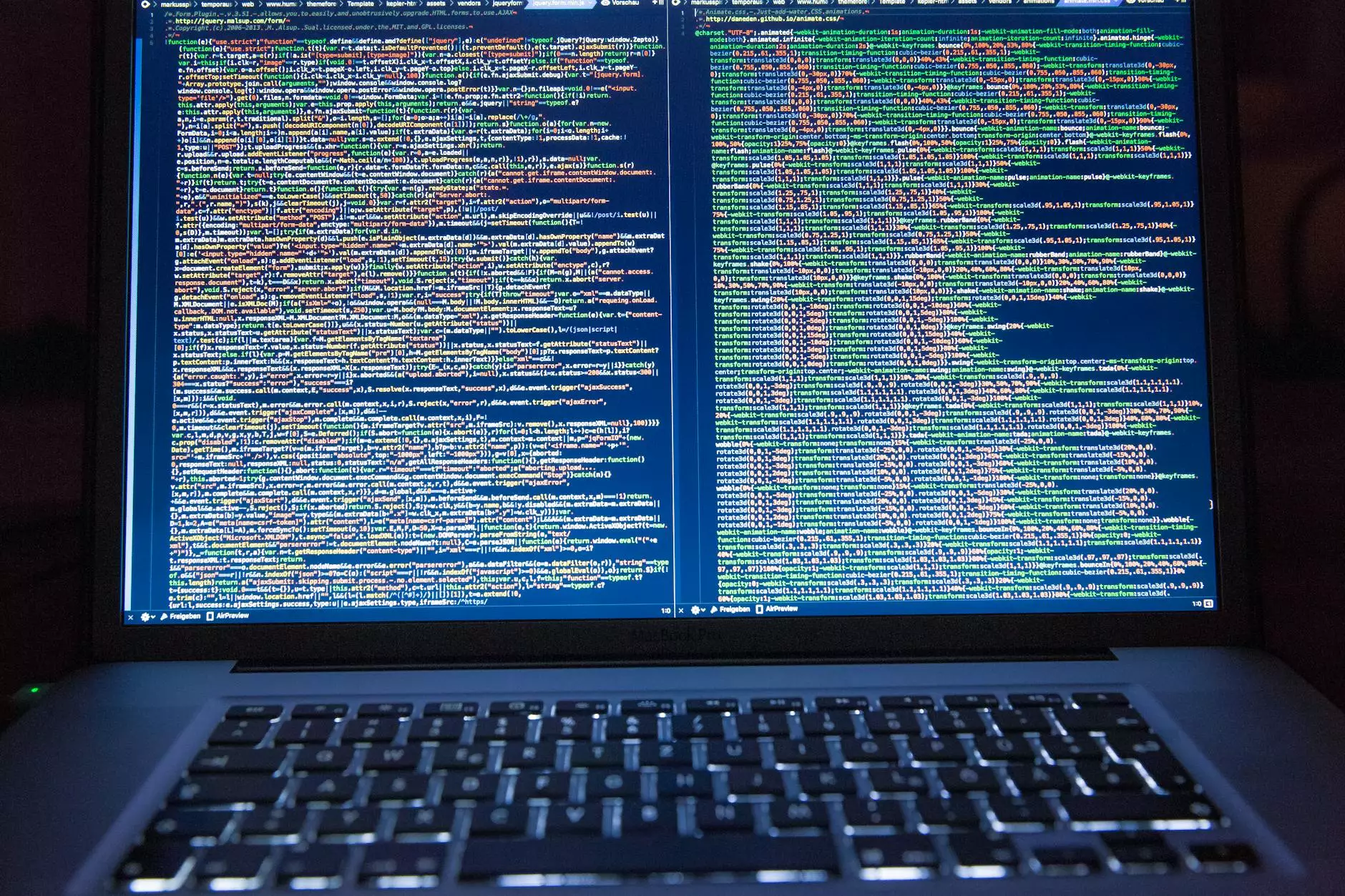
Introduction
Welcome to The Broad Life's comprehensive guide on how to identify and handle duplicate characters in a string while programming in C. Understanding how to work with duplicate characters is crucial in many programming tasks, so we have crafted this in-depth article to help you master this concept.
What Are Duplicate Characters in a String?
Before delving into the techniques for identifying duplicate characters in a string in C, let's clarify what duplicate characters actually are. Duplicate characters refer to the occurrence of the same character multiple times within a given string. Detecting and managing these duplicates is essential for various programming tasks and can significantly impact the efficiency and accuracy of your code.
Methods for Detecting Duplicate Characters
There are several approaches to identifying duplicate characters in a string in C. Below are some popular methods:
- Method 1: Using an Array - This method involves utilizing an array to store the frequency of each character in the string.
- Method 2: Sorting the String - By sorting the string, duplicate characters can be easily identified by comparing adjacent characters.
- Method 3: Hashing Technique - Implementing a hashing technique can expedite the detection of duplicate characters within a string.
Implementing Efficient Solutions
Efficiency is paramount when dealing with duplicate characters in a string. By choosing the right method and approach, you can enhance the performance of your C program. Consider the following tips:
- Opt for Data Structures: Utilize appropriate data structures such as arrays, sets, or maps to efficiently store and handle duplicate characters.
- Algorithm Optimization: Refine your algorithms to minimize time complexity and improve overall execution speed.
- Testing and Debugging: Thoroughly test your code to ensure accuracy and identify any potential issues related to duplicate characters.
Practical Examples and Code Snippets
To solidify your understanding of detecting duplicate characters in a string in C, let's explore some practical examples and code snippets:
#include int main() { char str[] = "duplicate characters in a string in c 0934225077"; int freq[256] = {0}; /* Your code implementation here */ return 0; }Conclusion
Congratulations! You have now gained valuable insights into effectively detecting and handling duplicate characters in a string in the C programming language. By mastering these techniques, you can optimize your code and enhance your programming skills. Stay tuned for more expert tips and guides from The Broad Life!